In this article you will know how to create Drag and Drop File Upload using HTML CSS and JavaScript. drag and drop file upload javascript you can create very easily if you know basic javascript. Earlier I have shared many more types of Drag and Drop elements and File Upload and Preview section. This is basically a drag and drop multiple file upload that I made with javascript.
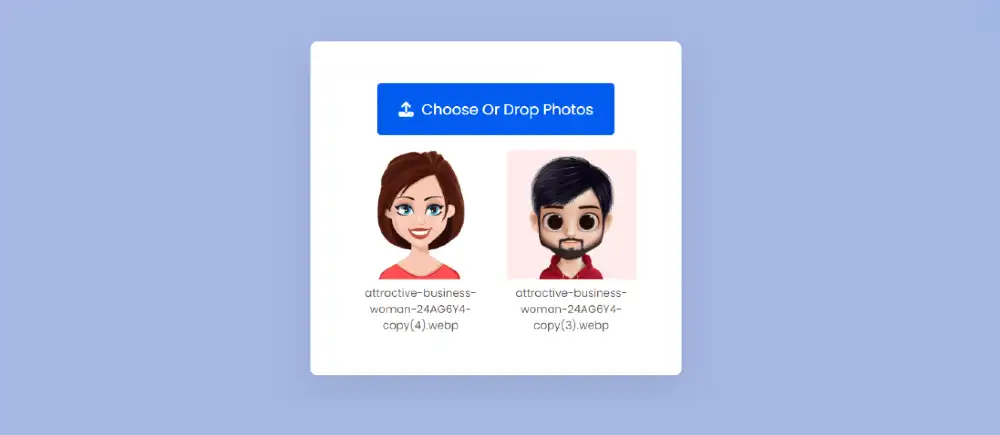
Now it’s time to create JavaScript Drag and Drop File Upload. Here I have created the basic structure by html. I designed it with css and activated this Drag & Drop or Browse – File upload with javascript.
20+ Free CSS confetti animations (Code+ Demo)
drag & drop file upload javascript
Drag and drop file upload in JavaScript refers to the ability to select one or more files using the drag and drop gesture, and then upload those files to a server.
This feature is typically implemented using JavaScript event listeners, such as “dragover” and “drop,” that detect when a file is being dragged over a specific element on the page and when the file is dropped onto that element. Within this project (drag and drop file upload javascript) you can upload the image by selecting it and you can also upload it by drag and drop. Also here you can upload multiple images.
See the Pen Drag and Drop File Upload with JavaScript by Ground Tutorial (@groundtutorial) on CodePen.
As you can see above this is a simple javascript drag and drop file upload project. Here I have created a small area or box. Inside that box is a button. You can select the image by clicking on that button or you can drag and drop the image into the box. Then the uploaded image can be seen in the preview box below.
How To Make A Drag and Drop File Uploader With JavaScript
Now if you want to build(How To Make A Drag-and-Drop File Uploader With javascript) this project then follow the step by step tutorial below. Here I have given you the necessary explanation and source code to create drag and drop file upload javascript. A bit more JavaScript is used here. But there is no reason to worry. I have explained each code step by step and tried to explain you completely why I used that line of code.
20+ CSS Play Button (Code + Demo)
Besides, I have given a preview after each step. Which will help you to know what kind of result will be seen after using that code.
Step 1: Basic Structure of Drag and Drop File Uploader
I have created the basic structure of this Drag and drop multiple file upload using the following html and css. All the information of that box can be seen. I used white for the background color of the box and some shadows around it.
* {
padding: 0;
margin: 0;
box-sizing: border-box;
font-family: "Poppins", sans-serif;
}
body {
background-color: #a4b7e3;
}
.container {
background-color: #ffffff;
width: 60%;
min-width: 27.5em;
padding: 3.12em 1.87em;
position: absolute;
transform: translate(-50%, -50%);
left: 50%;
top: 50%;
box-shadow: 0 1.25em 3.43em rgba(0, 0, 0, 0.08);
border-radius: 0.5em;
}

Step 2: Create a file upload button
Now an input button is created to upload the file in drag and drop file upload javascript. I have created this button using HTML’s input function. For uploading image only I used accept=”image/*” which basically will help to upload image file only.
input[type="file"] {
display: none;
}
label {
display: block;
position: relative;
background-color: #025bee;
color: #ffffff;
font-size: 1.1em;
text-align: center;
width: 16em;
padding: 1em 0;
border-radius: 0.3em;
margin: 0 auto 1em auto;
cursor: pointer;
}

Step 3: Create a place to preview the image
Now an area is created, in which the images can be viewed i.e. image preview area is created. How images are displayed is determined by css.
#image-display {
position: relative;
width: 90%;
margin: 0 auto;
display: flex;
justify-content: space-evenly;
gap: 1.25em;
flex-wrap: wrap;
}
#image-display figure {
width: 45%;
}
#image-display img {
width: 100%;
}
#image-display figcaption {
font-size: 0.8em;
text-align: center;
color: #5a5861;
}
.active {
border: 0.2em dashed #025bee;
}
#error {
text-align: center;
color: #ff3030;
}
Step 4: Enable the drag and drop multiple files uploader
Now it’s time to implement this drag and drop file uploader using javascript. Here I have given the necessary explanation, hope you will not have any difficulty in understanding.
These lines of code are assigning variables to different elements on a webpage by their ID to active Drag And Drop File Uploader in javascript. The variables are:
uploadButton
is assigned to the element with the ID “upload-button”chosenImage
is assigned to the element with the ID “chosen-image”fileName
is assigned to the element with the ID “file-name”container
is assigned to the first element with the class “container”error
is assigned to the element with the ID “error”imageDisplay
is assigned to the element with the ID “image-display”
It appears that these variables will be used to manipulate these elements and their properties in later JavaScript code.
let uploadButton = document.getElementById("upload-button");
let chosenImage = document.getElementById("chosen-image");
let fileName = document.getElementById("file-name");
let container = document.querySelector(".container");
let error = document.getElementById("error");
let imageDisplay = document.getElementById("image-display");
This code defines a function fileHandler()
that takes in three parameters: file
, name
, and type
. The function checks if the file is of type image by checking the first part of the type string before “/” if it is not of type image it sets the innerText of the error element to “Please upload an image file” and returns false.
If the file is an image, it sets the innerText of the error element to an empty string and creates a new FileReader object, which reads the contents of the file as a Data URL.
When the file has been read, the onloadend
event is triggered, at this point it creates an imageContainer
element and an img
element, sets the source of the img
element to the result of the file reader, appends the img
element to the imageContainer
element, and then appends a figcaption
element containing the file name to the imageContainer
element. Finally, it appends the imageContainer
element to the imageDisplay
element.
const fileHandler = (file, name, type) => {
if (type.split("/")[0] !== "image") {
//File Type Error
error.innerText = "Please upload an image file";
return false;
}
error.innerText = "";
let reader = new FileReader();
reader.readAsDataURL(file);
reader.onloadend = () => {
//image and file name
let imageContainer = document.createElement("figure");
let img = document.createElement("img");
img.src = reader.result;
imageContainer.appendChild(img);
imageContainer.innerHTML += `${name} `;
imageDisplay.appendChild(imageContainer);
};
};
Now Drag and Drop File Upload project upload button should be activated. This code attaches an event listener to the uploadButton
element that listens for a “change” event. When the event is fired, the function inside the event listener is executed. The function clears the content of the imageDisplay
element by setting its innerHTML to an empty string.
Then it creates an array of the files from the uploadButton
element, and for each file in that array, it calls the fileHandler()
function and passes in the file
, the file.name
, and the file.type
as the arguments.
This code is used to handle the event of a user selecting and uploading one or more image files, displaying each image file and its name on the page by calling the fileHandler
function for each file.
//Upload Button
uploadButton.addEventListener("change", () => {
imageDisplay.innerHTML = "";
Array.from(uploadButton.files).forEach((file) => {
fileHandler(file, file.name, file.type);
});
});
Now you need to define what happens when you drag the file into the Simple javascript Drag and Drop File Upload box. This code attaches an event listener to the container
element that listens for a “dragenter” event. When the event is fired, the function inside the event listener is executed. The function calls preventDefault()
and stopPropagation()
on the event object (e
) to prevent the default browser behavior and stop the event from propagating.
Then it adds a class “active” to the container
element’s classList. This code is probably used to handle the event of a user dragging an item over the container
element, indicating that the container is a valid drop target.
Circular Progress Bar using only HTML and CSS
The class “active” is added to the container which can be used to style it and give visual feedback that the container is active and ready to accept the dragged item.
container.addEventListener(
"dragenter",
(e) => {
e.preventDefault();
e.stopPropagation();
container.classList.add("active");
},
false
);
Now you need to determine what happens if you drag leave within the HTML drag drop upload area. This code attaches an event listener to the container
element that listens for a “dragleave” event. When the event is fired, the function inside the event listener is executed. The function calls preventDefault()
and stopPropagation()
on the event object (e
) to prevent the default browser behavior and stop the event from propagating.
Then it removes the class “active” from the container
element’s classList. This code is probably used to handle the event of a user dragging an item out of the container
element, indicating that the container is no longer a valid drop target.
The class “active” is removed from the container, it can be used to style it and give visual feedback that the container is no longer active and no longer ready to accept the dragged item.
container.addEventListener(
"dragleave",
(e) => {
e.preventDefault();
e.stopPropagation();
container.classList.remove("active");
},
false
);
Upload files with Drag and Drop to determine what happens when dragover. This code attaches an event listener to the container
element that listens for a “dragover” event. When the event is fired, the function inside the event listener is executed. The function calls preventDefault()
and stopPropagation()
on the event object (e
) to prevent the default browser behavior and stop the event from propagating.
container.addEventListener(
"dragover",
(e) => {
e.preventDefault();
e.stopPropagation();
container.classList.add("active");
},
false
);
This code attaches an event listener to the container
element that listens for a “drop” event in drag and drop file upload javascript. When the event is fired, the function inside the event listener is executed. The function calls preventDefault()
and stopPropagation()
on the event object (e
) to prevent the default browser behavior and stop the event from propagating.
Then it removes the class “active” from the container
element’s classList. It then creates a variable draggedData
which holds the dataTransfer object of the event, which contains the data that was dropped onto the element. It then creates a variable files
which holds the file object from the draggedData
object. It clears the content of the imageDisplay
element by setting its innerHTML to an empty string.
Then it creates an array of the files and for each file in that array, it calls the fileHandler()
function and passes in the file
, the file.name
, and the file.type
as the arguments. This code is used to handle the event of a user dropping one or more files on to the container
element, which then calls the fileHandler
function to display the image and its name on the page.
container.addEventListener(
"drop",
(e) => {
e.preventDefault();
e.stopPropagation();
container.classList.remove("active");
let draggedData = e.dataTransfer;
let files = draggedData.files;
imageDisplay.innerHTML = "";
Array.from(files).forEach((file) => {
fileHandler(file, file.name, file.type);
});
},
false
);
Now it is determined that every time the page is loaded all the information contained in the Drag and Drop File Upload with HTML CSS JavaScript will be removed. This code assigns an anonymous function to the onload
property of the window
object, which is fired when the page has finished loading. The function sets the innerText
of the error
element to an empty string, effectively clearing any pre-existing text.
window.onload = () => {
error.innerText = "";
};
Hope you got to know from above tutorial how I created this project(Drag and Drop File Upload Using HTML,CSS & JavaScript). I have already shared many more JavaScript tutorials with you.
Be sure to comment how you liked this Drag and Drop File Upload JavaScript tutorial.
Yes, it is possible to implement drag and drop functionality for multiple files using JavaScript, HTML, and CSS. The code you provided is an example of how this can be done. The key elements in this implementation are:
- HTML elements to create the container for the drag and drop, the upload button and the display area for the selected files.
- JavaScript event listeners attached to the container to handle the drag and drop events (dragenter, dragleave, dragover, drop) and the upload button change event.
- JavaScript functions to handle the files that are dropped or selected through the upload button, such as the
fileHandler()
function which reads the file, creates an image element and displays it along with its name in the imageDisplay element. - CSS to style the container and give visual feedback to the user when a file is being dragged over the container.
To make a drag and drop file upload in JavaScript, you can follow these steps:
- Create an HTML container element that will act as the drop zone for the files.
- Attach event listeners to the container element for the drag events (dragenter, dragleave, dragover) and the drop event. These event listeners should handle the visual feedback for the user and prevent the default browser behavior.
- In the drop event listener, retrieve the files that were dropped by accessing the
dataTransfer
object on the event and then retrieve thefiles
property. - Iterate through the files and handle them as needed, for example, by calling a function that reads the file and displays it on the page.
Yes, it is possible to implement drag and drop functionality for files using only JavaScript, without using any libraries or frameworks. Here’s an example of how you can do this:
Create an HTML container element that will act as the drop zone for the files.
Attach event listeners to the container element for the drag events (dragenter, dragleave, dragover) and the drop event. These event listeners should handle the visual feedback for the user and prevent the default browser behavior.