Do you want to create Show and hide password using JavaScript?
In this article you will know how to create show password eye icon javascript. This type of toggle password visibility eye can be found in various login or registration forms. Where the user can hide and show them after inputting the password.
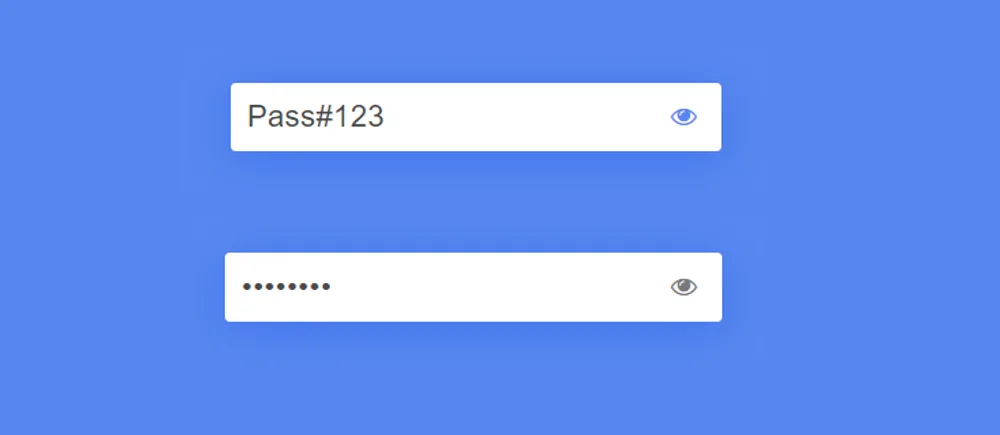
show/hide password eye icon A simple design that you can easily create with JavaScript. If you have basic idea about javascript then you can create this project very easily.
JavaScript Show-Hide Password Toggle
Showing and hiding passwords in a web application is a common requirement for many websites that require users to create accounts or login. This(How to Toggle Password Visibility in JavaScript) can be accomplished by using JavaScript and HTML.
The simplest way to show and hide passwords using javascript is to use two buttons. The first button will show the password and the second button will hide it. To implement this functionality, you can add an event listener to each button that will change the type of the input field that holds the password.
Here’s an example of how you can show and hide a password using JavaScript:
See the Pen Untitled by Ground Tutorial (@groundtutorial) on CodePen.
Hopefully from the above preview you have learned how this JavaScript Toggle Show and Hide Password works. As you can see above I have created a small input box within a web page. I used css to design that input box a bit.
In that input box there is an icon to show hide password. Clicking on this icon will show and hide your inputted password.
How to Toggle Show and Hide Password in Javascript
Now if you want to make it then follow the tutorial below. First the basic structure of input password show hide using html is created. Then added input box and eye icon.
Then designed this js show password using css and finally implemented it with javascript. If you only need the source code, use the download button below the article.
1. HTML code for input password show hide
First I created all the basic structure using the following html codes. Very little JavaScript is used here. To create this project first I created a box or area.
Then I created the input box using html’s input function. Then added an eye icon here. You can add any other icon instead of this icon. Not only that you can use text instead of icons.
2. CSS code for Show-Hide Password
Now I have designed some basic web page using some amount of CSS. Here I have used blue background color on the webpage. Besides, position: absolute, transform: translate(-50%,-50%) is used to place the Show and Hide password field in the middle of the page.
body{
background-color: #5887ef;
padding: 0;
margin: 0;
}
.wrapper{
position: absolute;
transform: translate(-50%,-50%);
top: 50%;
left: 50%;
width: 300px;
}
Now we need to design from the input box. Input box width: 300px is used.
input{
box-sizing: border-box;
width: 100%;
font-size: 18px;
border: none;
padding: 10px 10px;
border-radius: 3px;
font-family: 'Poppins',sans-serif;
color: #4a4a4a;
letter-spacing: 0.5px;
box-shadow: 0 5px 30px rgba(22,89,233,0.4);
}
::placeholder{
color: #9a9a9a;
font-weight: 400;
}
Now designed the eye icon used for Toggle password visibility. If you’re using text instead of icons, you’ll need to change the CSS here accordingly.
span{
position: absolute;
right: 15px;
transform: translate(0,-50%);
top: 50%;
cursor: pointer;
}
.fa{
font-size: 20px;
color: #7a797e;
}
3. JavaScript for Toggle Show Hide Password
Now it’s time to activate the Show-Hide Password Toggle with JavaScript. Very little javascript is used here so you won’t have any difficulty in understanding.
Below I have given all the code and explanation how this javascript will make this project (How to Toggle Show and Hide Password in Javascript) work.
var state= false;
function toggle(){
if(state){
document.getElementById("password").setAttribute("type","password");
document.getElementById("eye").style.color='#7a797e';
state = false;
}
else{
document.getElementById("password").setAttribute("type","text");
document.getElementById("eye").style.color='#5887ef';
state = true;
}
}
This is a JavaScript function that toggles the visibility of a password input field. When the function is called, it checks the current state of the state
variable. If the state
is true
, it sets the type attribute of the password input field to “password” and changes the color of an element with the ID “eye” to ‘#7a797e’.
If the state
is false
, it sets the type attribute of the password input field to “text” and changes the color of the “eye” element to ‘#5887ef’. After the changes are made, the state
variable is updated to its opposite value.
Hope from this tutorial you know how I created this Javascript Show-Hide Password Toggle. If there is any problem while creating this html Toggle Password Visibility then you can comment me.
Here is an example of how to show and hide a password in JavaScript:
var state = false;
function toggle() {
if (state) {
document.getElementById("password").setAttribute("type", "password");
state = false;
} else {
document.getElementById("password").setAttribute("type", "text");
state = true;
}
}
In this example, we use a state
variable to keep track of whether the password is currently shown or hidden. The toggle
function changes the type attribute of the password input field with an ID of “password” from “password” to “text” or vice versa, depending on the current state.
The state
variable is updated after each toggle to keep track of the current state. To use this in a HTML page, you would need to add an input field with the id
attribute set to “password” and a button or other element that calls the toggle
function when it is clicked.
Here’s a complete HTML, CSS, and JavaScript example of how to create a toggle button to show and hide a password input field:
<div>
<input type="password" id="password">
<button id="toggleBtn" onclick="toggle()">Show </button>
</div>
var state = false;
function toggle() {
if (state) {
document.getElementById("password").setAttribute("type", "password");
document.getElementById("toggleBtn").innerHTML = "Show";
state = false;
} else {
document.getElementById("password").setAttribute("type", "text");
document.getElementById("toggleBtn").innerHTML = "Hide";
state = true;
}
}
div {
display: flex;
align-items: center;
}
input[type="password"] {
margin-right: 10px;
}
button {
background-color: lightgray;
border: none;
padding: 5px 10px;
}
This will create a password input field with a toggle button next to it. The JavaScript function toggle
changes the type attribute of the password input field from “password” to “text” or vice versa, depending on the current state.
The text on the button is updated to reflect the current state (either “Show” or “Hide”). The CSS is optional and is used to style the appearance of the input field and button.
Here’s an example of how to show and hide a password input field using an eye icon in HTML:
<div class="password-container">
<input type="password" id="password">
<span class="show-password" onclick="toggle()">
<i class="fas fa-eye" id="eye"></i>
</span>
</div>
var state = false;
function toggle() {
if (state) {
document.getElementById("password").setAttribute("type", "password");
document.getElementById("eye").classList.remove("fa-eye-slash");
document.getElementById("eye").classList.add("fa-eye");
state = false;
} else {
document.getElementById("password").setAttribute("type", "text");
document.getElementById("eye").classList.remove("fa-eye");
document.getElementById("eye").classList.add("fa-eye-slash");
state = true;
}
}
.password-container {
position: relative;
}
input[type="password"] {
padding-right: 40px;
}
.show-password {
position: absolute;
right: 10px;
top: 50%;
transform: translateY(-50%);
cursor: pointer;
}
.fa-eye, .fa-eye-slash {
font-size: 18px;
}
This example uses Font Awesome icons to display an eye icon to toggle the visibility of the password. The JavaScript toggle
function changes the type attribute of the password input field and updates the eye icon accordingly.
The CSS is optional and is used to position the eye icon and style the appearance of the input field. To use this example, you need to include Font Awesome icons in your project.