Introduction :
This project is a simple yet functional notes-taking web application built using HTML, CSS, and JavaScript. The app allows users to create, display, and delete notes. It also includes a search feature to filter notes based on user input. The notes are stored in the browser’s localStorage
, ensuring that they persist even after the browser is closed.
It is a straightforward yet powerful web application designed to help users capture and manage their notes efficiently. Built using foundational web technologies—HTML for structure, CSS for styling, and JavaScript for functionality—this application provides a seamless experience for note-taking.
Upon loading the app, users are greeted with a clean and intuitive interface where they can quickly add new notes, view existing ones, and delete notes they no longer need. Each note is stored in the browser’s localStorage
, ensuring that users’ notes persist even after the browser is closed or refreshed. This local storage capability makes the app highly reliable and practical for everyday use, as it eliminates the need for server-side storage and enhances data privacy.
The user interface is designed with simplicity and usability in mind. A prominent text area allows users to enter their notes easily, and a clearly labeled button facilitates the saving of these notes. Once saved, notes are displayed in an organized manner, with each note encapsulated within a card layout. This not only enhances readability but also allows users to manage their notes effectively.
In addition to the basic add and delete functionalities, the app includes a search feature that enables users to filter through their notes based on keywords. This feature is particularly useful for users who need to quickly locate specific information within a large collection of notes.
The Notes Taking App demonstrates the effective use of JavaScript to manipulate the Document Object Model (DOM), handle events, and manage data storage in the browser. The project is an excellent example of how modern web development practices can be applied to create useful and user-friendly applications. It highlights the power of client-side storage and provides a solid foundation for further enhancements, such as categorization of notes, cloud storage integration, or additional user authentication features.
Overall, this Notes Taking App not only serves a practical purpose but also illustrates key web development concepts, making it an ideal project for both end users and developers looking to deepen their understanding of web technologies.
Explanation :
HTML Structure
The HTML structure forms the backbone of the Notes Taking App, defining the layout and elements that users interact with. It consists of a main container, input fields for adding notes, and a section for displaying the saved notes.
Header Section: The header includes a
<h1>
element that serves as the main title of the app, providing a clear indication to the user about the purpose of the application.Note Addition Section:
- Card Component: This section is enclosed within a card component for a clean and organized appearance. The card component includes:
- Title: A secondary heading (
<h5>
) that prompts the user to add a new note. - Text Area: A large text area input where users can type their notes. This input is identified by the
id
attributeaddTxt
, making it easy to reference in the JavaScript code. - Button: A primary button labeled “Add Note” which, when clicked, triggers the function to save the note. The button is identified by the
id
attributeaddBtn
.
- Title: A secondary heading (
- Card Component: This section is enclosed within a card component for a clean and organized appearance. The card component includes:
Notes Display Section:
- Headers: Another set of headers (
<h1>
) to distinguish between the note addition section and the display section. - Notes Container: A
<div>
element with theid
notes
, which serves as a container for all the notes dynamically generated by the JavaScript code. This container starts off empty and gets populated with note cards.
- Headers: Another set of headers (
CSS Styling
The CSS file provides the visual styling for the application, ensuring it looks modern and responsive.
Body Styling: The body is styled to use a clean, sans-serif font and is centered using flexbox for a balanced layout on various screen sizes.
Container: The main container is set to a maximum width of 800px and is centered both vertically and horizontally. This ensures that the content does not stretch too wide on large screens and remains readable.
Card Component: The card component, used for both the note addition form and each note, has padding and margin set for spacing and includes rounded corners and a shadow to stand out visually.
Buttons: The primary button is styled with a distinctive color, padding, and border radius to make it visually appealing and easy to interact with.
Responsive Design: The layout is designed to be responsive, ensuring that it looks good on devices of all sizes, from mobile phones to desktop monitors.
JavaScript Logic
The JavaScript code is the functional core of the application, handling the logic for adding, displaying, deleting, and searching notes.
Initialization: Upon loading the app, the
showNotes()
function is called to display any existing notes stored inlocalStorage
. This ensures that users see their saved notes immediately without any additional action.Adding a Note:
- Event Listener: An event listener is attached to the “Add Note” button. When clicked, this triggers a function that reads the content of the text area.
- Storage Management: The function checks
localStorage
for existing notes. If none are found, it initializes an empty array. The new note is then added to this array, and the updated array is saved back tolocalStorage
. - UI Update: After saving the note, the text area is cleared, and
showNotes()
is called to refresh the displayed list of notes.
Displaying Notes:
- Retrieving Notes: The
showNotes()
function reads the notes fromlocalStorage
, parses the JSON string into an array, and iterates over it to create HTML content for each note. - Dynamic Content Creation: Each note is wrapped in a card component with a delete button. The generated HTML is then inserted into the
notes
container. - Empty State Handling: If no notes are found, a placeholder message is displayed to prompt the user to add new notes.
- Retrieving Notes: The
Deleting a Note:
- Delete Button: Each note card includes a delete button with an
onclick
attribute that calls thedeleteNote()
function with the specific note’s index. - Updating Storage: The
deleteNote()
function removes the note at the given index from the array and updateslocalStorage
. It then callsshowNotes()
to refresh the displayed notes.
- Delete Button: Each note card includes a delete button with an
Searching Notes:
- Search Input: An event listener is attached to the search input field. As the user types, this triggers a function that filters the displayed notes.
- Filtering Logic: The function reads the input value, converts it to lowercase for case-insensitive comparison, and iterates over the note cards. Notes that match the search term remain visible, while others are hidden.
Purpose of Functions
showNotes(): This function is crucial for displaying the current state of the notes. It reads from
localStorage
, constructs the HTML for each note, and updates the UI. It ensures that any changes to the notes (adding or deleting) are immediately reflected in the UI.deleteNote(): This function handles the deletion of notes. It updates the array of notes stored in
localStorage
and refreshes the UI to remove the deleted note. This function enhances user experience by providing a way to manage and clean up notes.Search Input Event Listener: This feature allows users to quickly filter and find specific notes. By dynamically hiding and showing notes based on the search term, it improves the usability of the app, especially when dealing with a large number of notes.
Conclusion
This detailed explanation covers the core components and functionality of the Notes Taking App. It highlights how HTML, CSS, and JavaScript work together to create a cohesive and interactive web application. The use of localStorage
for persistent data storage ensures that notes are not lost between sessions, making the app both practical and reliable for everyday use. The clean and responsive design, combined with intuitive functionalities like adding, deleting, and searching notes, provides a robust user experience.
SOURCE CODE :
HTML (index.html)
Notes taking app
Record your notes here
Add a note
Your Notes
CSS (style.css)
body {
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
display: flex;
justify-content: center;
}
.navbar {
background-color: #343a40;
padding: 1rem;
}
.navbar-brand {
color: #ffffff;
font-size: 1.5rem;
font-weight: bold;
}
.navbar-toggler {
background-color: #ffffff;
}
.navbar-toggler-icon {
color: #343a40;
}
.navbar-nav {
margin-top: 10px;
}
.nav-item {
margin-right: 10px;
}
.form-inline {
display: flex;
align-items: center;
}
.form-control {
margin-right: 10px;
}
.container {
max-width: 800px;
}
.card {
margin-top: 20px;
}
.card-body {
padding: 20px;
}
.btn-primary {
background-color: #007bff;
color: #ffffff;
border: none;
padding: .8rem;
border-radius: .3rem;
}
.btn-outline-success {
color: #28a745;
border-color: #28a745;
}
hr {
border: 1px solid #343a40;
margin: 20px 0;
}
h1 {
font-size: 2rem;
opacity: .6;
}
.container {
display: flex;
flex-direction: column;
justify-content: center;
align-items: center;
width: 100%;
background-color: #eeeeee;
}
textarea {
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
font-size: 1rem;
padding: .5rem;
}
JavaScript (index.js)
console.log("Welcome to notes app. This is app.js");
showNotes();
// If user adds a note, add it to the localStorage
let addBtn = document.getElementById("addBtn");
addBtn.addEventListener("click", function (e) {
let addTxt = document.getElementById("addTxt");
let notes = localStorage.getItem("notes");
if (notes == null) {
notesObj = [];
} else {
notesObj = JSON.parse(notes);
}
notesObj.push(addTxt.value);
localStorage.setItem("notes", JSON.stringify(notesObj));
addTxt.value = "";
showNotes();
});
// Function to show elements from localStorage
function showNotes() {
let notes = localStorage.getItem("notes");
if (notes == null) {
notesObj = [];
} else {
notesObj = JSON.parse(notes);
}
let html = "";
notesObj.forEach(function (element, index) {
html += `
Note ${index + 1}
${element}
`;
});
let notesElm = document.getElementById("notes");
if (notesObj.length != 0) {
notesElm.innerHTML = html;
} else {
notesElm.innerHTML = `Nothing to show! Use "Add a Note" section above to add notes.`;
}
}
// Function to delete a note
function deleteNote(index) {
// console.log("I am deleting", index);
let notes = localStorage.getItem("notes");
if (notes == null) {
notesObj = [];
} else {
notesObj = JSON.parse(notes);
}
notesObj.splice(index, 1);
localStorage.setItem("notes", JSON.stringify(notesObj));
showNotes();
}
let search = document.getElementById('searchTxt');
search.addEventListener("input", function () {
let inputVal = search.value.toLowerCase();
// console.log('Input event fired!', inputVal);
let noteCards = document.getElementsByClassName('noteCard');
Array.from(noteCards).forEach(function (element) {
let cardTxt = element.getElementsByTagName("p")[0].innerText;
if (cardTxt.includes(inputVal)) {
element.style.display = "block";
}
else {
element.style.display = "none";
}
// console.log(cardTxt);
})
})
OUTPUT :
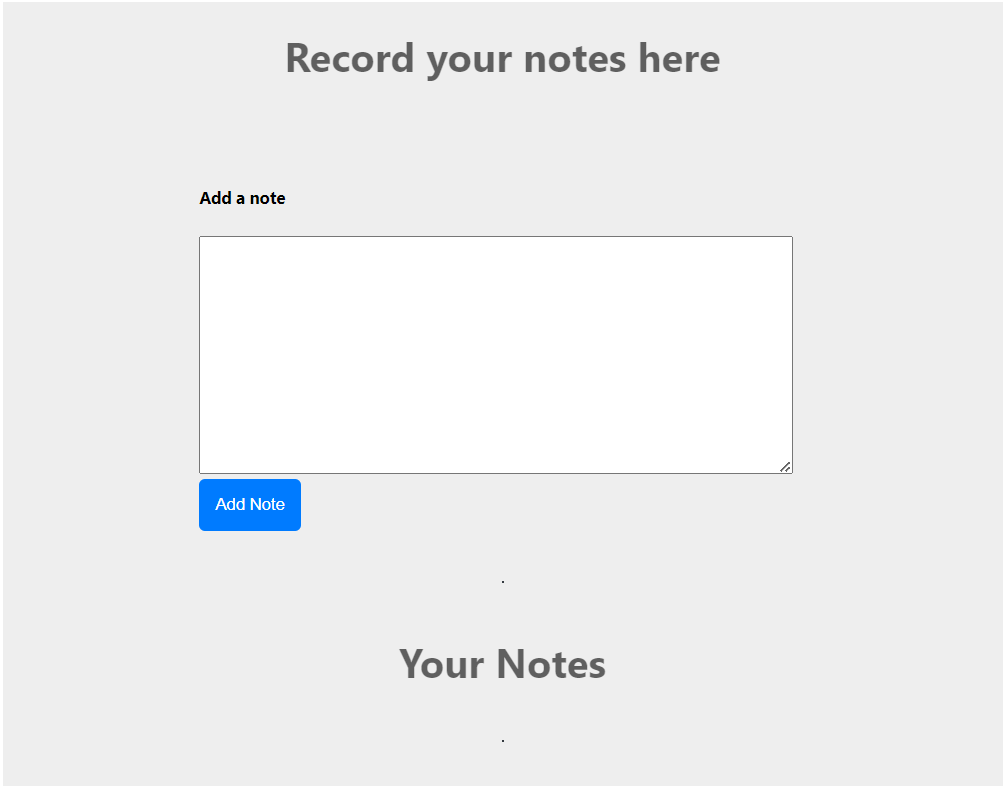