Introduction :
This News App is a web application designed to deliver news articles to users based on specific categories and search queries. Built using HTML, CSS, and JavaScript, the app provides a user-friendly interface to fetch and display news articles dynamically from the NewsAPI. Users can navigate through different news categories and search for specific news topics seamlessly.
It is a sophisticated web application crafted to deliver a seamless and engaging news browsing experience. It harnesses the power of HTML, CSS, and JavaScript to create a user-centric platform for accessing the latest news articles across various categories. With its intuitive interface and dynamic content delivery, the News App redefines how users stay informed in today’s fast-paced world.
Purpose and Scope
At its core, the News App aims to address the evolving needs of modern news consumers. By aggregating news from diverse sources and categories, it empowers users to explore a wide range of topics tailored to their interests. Whether it’s finance, sports, technology, or global affairs, the app provides a comprehensive selection of articles curated in real-time.
User Experience Design
A pivotal aspect of the News App is its user experience (UX) design. Every element, from the navigation bar to the layout of news cards, is meticulously crafted to optimize usability and visual appeal. Intuitive navigation features, such as clickable category links and a responsive search bar, ensure that users can effortlessly find the information they seek. The design’s responsiveness adapts seamlessly to different devices and screen sizes, guaranteeing a consistent and enjoyable experience across platforms.
Dynamic Content Delivery
Central to the News App’s functionality is its ability to fetch and display news articles dynamically. Leveraging the NewsAPI, the app retrieves up-to-date content based on user interactions, such as category selections and search queries. Through asynchronous JavaScript requests, the app seamlessly integrates external data, providing users with a continuous stream of fresh news articles without page reloads.
Personalization and Interactivity
Recognizing the importance of personalization, the News App empowers users to tailor their news consumption experience. By selecting preferred categories and conducting targeted searches, users can curate their news feed to reflect their specific interests and preferences. Additionally, interactive features like clickable news cards and real-time updates foster engagement and encourage exploration within the app’s ecosystem.
Conclusion
In essence, the News App represents a fusion of cutting-edge technology and user-centric design principles. It not only facilitates access to timely news content but also elevates the overall browsing experience through intuitive interface design and dynamic content delivery. As the digital landscape continues to evolve, the News App stands as a testament to innovation in news consumption, providing users with a gateway to the vast and ever-changing world of information.
Explanation :
HTML Structure
The HTML structure serves as the foundation of the News App, defining its layout and content hierarchy. Let’s break down its components:
Navigation Bar: Positioned at the top of the page, the navigation bar contains links to different news categories and a search bar. Each category link triggers a JavaScript function when clicked, initiating the process of fetching news articles related to the selected category.
Main Content Area: This section, encapsulated within the
<main>
tag, is where the news articles are displayed. It consists of a container (<div id="cards-container">
) that dynamically populates with news cards based on the fetched data.News Card Template: Defined within a
<template>
tag, the news card template serves as a blueprint for individual news cards. It contains placeholders for the news image, title, source, and description. JavaScript dynamically fills these placeholders with actual data fetched from the NewsAPI.
CSS Styling
The CSS stylesheet is responsible for styling the HTML elements, enhancing the visual appeal and user experience of the app. Here’s how it accomplishes this:
Global Styles: Resets default styles, sets font families, and applies basic styling to ensure consistency across different browsers and devices.
Navigation Bar Styling: Styles the navigation bar, including the appearance of navigation links, search bar, and overall layout. It also defines hover effects to provide visual feedback when users interact with the navigation elements.
Main Content Area Styling: Defines the layout and appearance of news cards within the main content area. This includes setting the dimensions, applying box shadows, and defining transitions for hover effects.
News Card Styling: Specifies the styles for individual news cards, including the appearance of the card header, image, title, source, and description. It also defines hover effects to enhance interactivity and engagement.
JavaScript Logic
The JavaScript file contains the logic and functionality that drive the behavior of the News App. Let’s explore its key components:
API Configuration: Defines the API key and base URL for the NewsAPI, which is used to fetch news data.
Event Listeners: Listens for specific events such as window load, navigation item clicks, and search button clicks. These events trigger functions to fetch news data and update the UI accordingly.
Fetch News Function: Sends asynchronous requests to the NewsAPI to fetch news articles based on a specified query (category or search term). Upon receiving a response, it parses the data and calls another function to bind the data to the UI.
Bind Data Function: Receives the fetched news articles and dynamically populates the news card template with the corresponding data. It then appends these populated cards to the main content area for display.
Helper Functions: Various helper functions are included to assist in filling data into news cards, handling navigation item clicks, and updating the active state of navigation links.
Purpose of Functions
Each function within the JavaScript file serves a specific purpose in facilitating the functionality and interactivity of the News App:
Fetch News Function: Retrieves news articles from the NewsAPI based on user input (category or search term).
Bind Data Function: Binds the fetched news data to the HTML template, dynamically generating news cards for display.
Helper Functions: Assist in handling user interactions (such as navigation item clicks and search button clicks), updating the UI accordingly, and maintaining the app’s state (e.g., tracking the active navigation item).
By modularizing the code into functions, the JavaScript file promotes maintainability, readability, and scalability, making it easier to debug, enhance, and extend the app’s functionality in the future.
SOURCE CODE :
HTML (index.html)
News App
This is the Title
End Gadget 26/08/2023
Lorem, ipsum dolor sit amet consectetur adipisicing elit. Recusandae
saepe quis voluptatum quisquam vitae doloremque facilis molestias quae ratione cumque!
CSS (style.css)
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@500&family=Roboto:wght@500&display=swap");
* {
padding: 0;
margin: 0;
box-sizing: border-box;
}
body {
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
background-color: #c3c3c3;
}
p {
font-family: "Roboto", sans-serif;
opacity: .8;
line-height: 1.4rem;
}
a {
text-decoration: none;
}
ul {
list-style: none;
}
.flex {
display: flex;
align-items: center;
}
.container {
max-width: 1180px;
margin-inline: auto;
overflow: hidden;
}
nav {
background-color: #484e52;
color: white;
position: fixed;
top: 0;
z-index: 99;
left: 0;
right: 0;
}
.main-nav {
justify-content: space-between;
padding-block: 8px;
}
.nav-links ul {
gap: 16px;
}
.hover-link {
cursor: pointer;
}
.nav-item.active {
color: var(--accent-color);
}
.search-bar {
height: 32px;
gap: 8px;
}
.news-input {
width: 200px;
height: 100%;
padding-inline: 12px;
border-radius: 4px;
border: 2px solid #bbd0e2;
font-family: "Roboto", sans-serif;
}
.search-button {
background-color: #208c5b;
color: white;
padding: 8px 24px;
border: none;
border-radius: 4px;
cursor: pointer;
font-family: "Roboto", sans-serif;
}
main {
padding-block: 20px;
margin-top: 80px;
}
.cards-container {
justify-content: space-between;
flex-wrap: wrap;
row-gap: 20px;
align-items: start;
}
.card {
width: 360px;
min-height: 400px;
box-shadow: 0 0 4px #d4ecff;
border-radius: 4px;
cursor: pointer;
background-color: #fff;
overflow: hidden;
transition: all 0.3s ease;
}
.card:hover {
box-shadow: 1px 1px 8px #d4ecff;
background-color: #f9fdff;
transform: translateY(-2px);
}
.card-header img {
width: 100%;
height: 180px;
object-fit: cover;
}
.card-content {
padding: 12px;
}
.news-source {
margin-block: 12px;
}
.hover-link:hover {
color: #b9b9b9;
text-decoration: underline;
}
JavaScript (script.js)
const API_KEY = "df36e349a02b48d197f5b9d9f649ecb4";
const url = "https://newsapi.org/v2/everything?q=";
window.addEventListener("load", () => fetchNews("India"));
function reload() {
window.location.reload();
}
async function fetchNews(query) {
const res = await fetch(`${url}${query}&apiKey=${API_KEY}`);
const data = await res.json();
console.log(data)
bindData(data.articles);
}
function bindData(articles) {
const cardsContainer = document.getElementById("cards-container");
const newsCardTemplate = document.getElementById("template-news-card");
cardsContainer.innerHTML = "";
articles.forEach((article) => {
if (!article.urlToImage) return;
const cardClone = newsCardTemplate.content.cloneNode(true);
fillDataInCard(cardClone, article);
cardsContainer.appendChild(cardClone);
});
}
function fillDataInCard(cardClone, article) {
const newsImg = cardClone.querySelector("#news-img");
const newsTitle = cardClone.querySelector("#news-title");
const newsSource = cardClone.querySelector("#news-source");
const newsDesc = cardClone.querySelector("#news-desc");
newsImg.src = article.urlToImage;
newsTitle.innerHTML = article.title;
newsDesc.innerHTML = article.description;
const date = new Date(article.publishedAt).toLocaleString("en-US", {
timeZone: "Asia/Jakarta",
});
newsSource.innerHTML = `${article.source.name} · ${date}`;
cardClone.firstElementChild.addEventListener("click", () => {
window.open(article.url, "_blank");
});
}
let curSelectedNav = null;
function onNavItemClick(id) {
fetchNews(id);
const navItem = document.getElementById(id);
curSelectedNav?.classList.remove("active");
curSelectedNav = navItem;
curSelectedNav.classList.add("active");
}
const searchButton = document.getElementById("search-button");
const searchText = document.getElementById("search-text");
searchButton.addEventListener("click", () => {
const query = searchText.value;
if (!query) return;
fetchNews(query);
curSelectedNav?.classList.remove("active");
curSelectedNav = null;
});
OUTPUT :
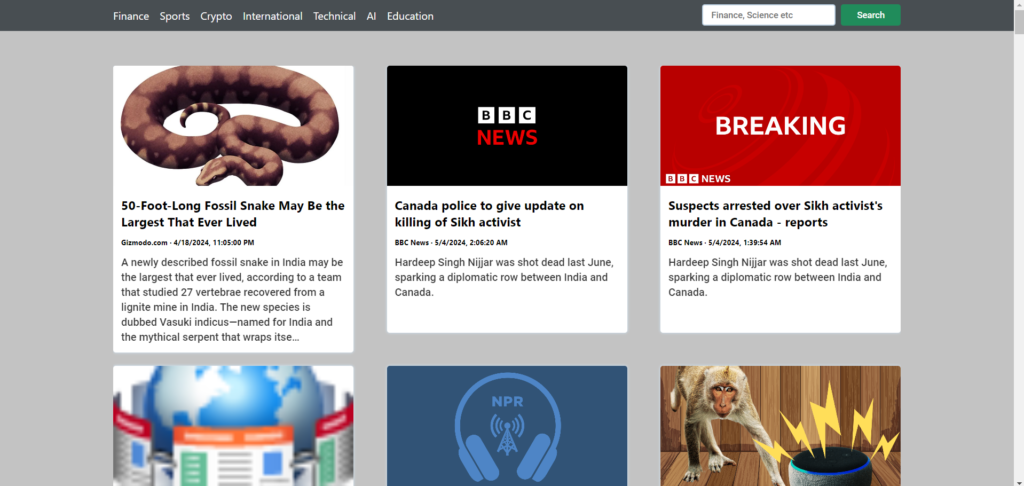