If you want to convert text to speech using javascript then this article. In this tutorial you will know how to create Text To Speech Converter using html css and javascript.
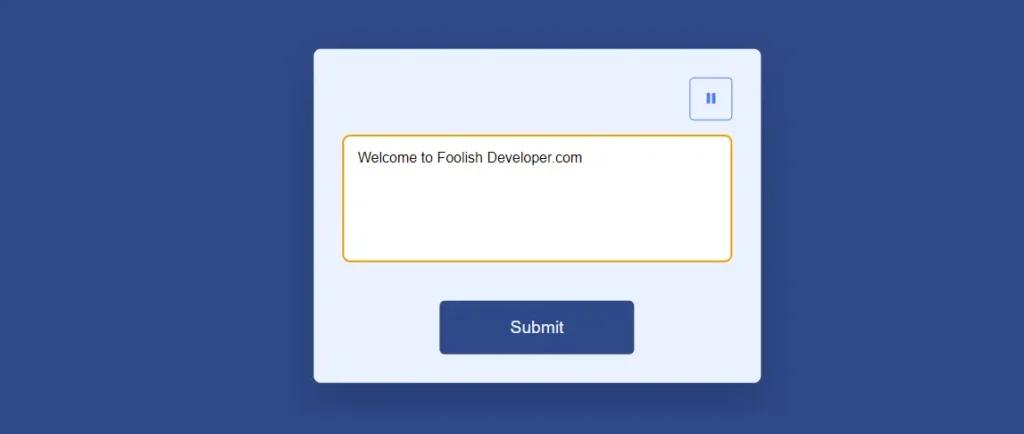
If you are a beginner and want to know about JavaScript then this tutorial will help you. This is a simple javascript text to speech converter where you input any text in the input box and hear it in audio form.
Text To Speech Converter JavaScript
We see javascript text to speech converter in many places. Not only this, this type of system is also used to convert the entire content into voice in different types of websites.
Earlier I shared another such design(How to Create Text To Speech Converter in HTML & JavaScript) which is more advanced. There you can choose the voice i.e. male and female. You can also change the tone of the voice.
But that design(How do I convert text to speech in JavaScript?) is quite difficult to make because the javascript used there is quite difficult. But this tutorial is best suited for beginners.
See the Pen Text To Speech Converter Javascript by Ground Tutorial (@groundtutorial) on CodePen.
As you can see I have colored the entire webpage blue and created a box above it. This box contains a textarea where you can input any text. Then there is a submit button at the bottom. Besides, there are buttons to play and stop audio.
Text To Speech Converter in HTML CSS & JavaScript
Now if you want to create Text To Speech Converter by Web APIs then this article is for you. HTML to create the basic structure of the project. Designed with CSS and implemented Text To Speech Converter with JavaScript.
If you want to download the complete source code, you can use the download button below the article. But it will be best if you follow the steps below.
1. Basic Structure of Text To Speech Converter
First I created a basic structure of this project using the code below. This basic structure has width: 90% and max-width: 37.5em. I also used the background color in the box.
* {
padding: 0;
margin: 0;
box-sizing: border-box;
font-family: "Poppins", sans-serif;
}
body {
height: 100vh;
background: #304988;
}
.container {
background-color: #ebf2ff;
width: 90%;
max-width: 37.5em;
position: absolute;
transform: translate(-50%, -50%);
left: 50%;
top: 50%;
border-radius: 0.5em;
padding: 2em;
box-shadow: 0 1.87em 3.75em rgba(2, 14, 44, 0.2);
}
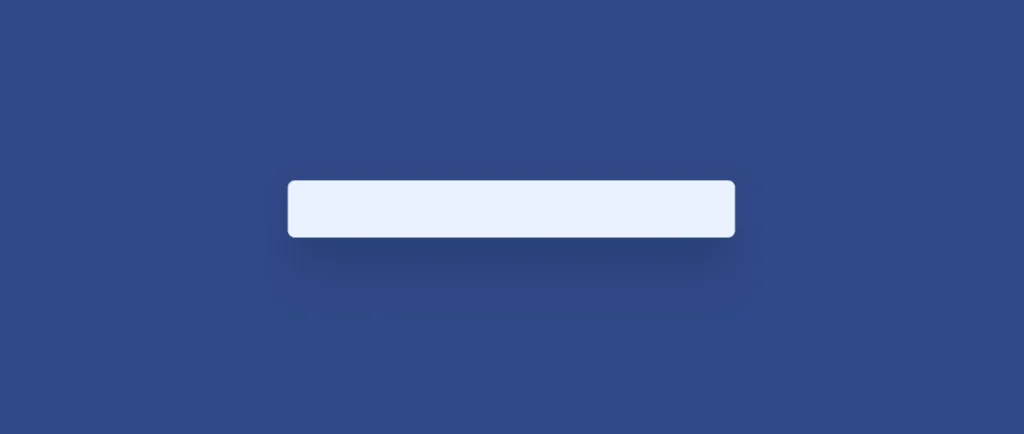
2. Create buttons to control Speech
Now in convert text to speech in JavaScript I will create control button. There are two control buttons one for play and one for stop.
.controls {
width: 100%;
display: flex;
justify-content: flex-end;
margin-bottom: 1em;
}
.controls button {
display: block;
width: 3em;
height: 3em;
font-size: 1em;
border-radius: 0.3em;
cursor: pointer;
color: #4c7df8;
border: 0.12em solid #4c7df8;
background-color: transparent;
}

3. Create text input area by textarea
Now create an input box using textarea. When you input something in that box, the text will be converted to voice. I used resize: none to fix the width: 100% and size of the textarea.
textarea {
font-size: 1em;
width: 100%;
border-radius: 0.5em;
padding: 1em;
resize: none;
border: 0.12em solid #040454;
}

4. Create a button within Text To Speech Converter
Now create a submit button in this Text to speech example. This button will basically work in your project. When you input the text in the input box, the text will be converted into voice by the submit button.
#submit {
font-size: 1.2em;
display: block;
width: 50%;
position: relative;
margin: auto;
padding: 1em;
border: none;
background: #304988;
color: #ebf2ff;
border-radius: 0.3em;
cursor: pointer;
margin-top: 2em;
}

5. Activate Text To Speech Converter by JavaScript
Here I have activated this Text To Speech Converter using Javascript. The JavaScript used here is quite simple. If you have a basic understanding of JavaScript, you can easily build it.
This code is using JavaScript to get references to elements on a webpage by their id values. It is also defining some variables such as “text”, “submitBtn”, “resumeBtn”, “pauseBtn”, and “audioMessage”. It is likely that these variables will be used later in the code to interact with the elements on the webpage.
It appears that the code is connecting to elements with the ids txt
, submit
, resume
, pause
. These elements likely correspond to some form of text input, a submit button, a resume button, and a pause button on the webpage, respectively.
The variable audioMessage
is being defined without any values, it used for audio related functionality in the later part of the code.
let text = document.getElementById("txt");
let submitBtn = document.getElementById("submit");
let resumeBtn = document.getElementById("resume");
let pauseBtn = document.getElementById("pause");
let audioMessage;
This code is using the addEventListener
method to attach an event handler to the “submitBtn” element. The event handler is a function that will be executed when the “click” event occurs on the “submitBtn” element.
The function inside the event listener is doing two things:
- It’s setting the
text
property of theaudioMessage
variable to the value of thetext
element, which is likely a text input on the webpage. - It’s using the
speak
method of thespeechSynthesis
object to start speaking the text of theaudioMessage
variable.
So, in summary, when the user clicks on the “submitBtn” element, the text in the “txt” element will be converted to speech by the browser’s speechSynthesis
API.
submitBtn.addEventListener("click", () => {
//set the text
audioMessage.text = text.value;
//speak the text
window.speechSynthesis.speak(audioMessage);
});
This code is using the addEventListener
method to attach an event handler to the “resumeBtn” element. The event handler is a function that will be executed when the “click” event occurs on the “resumeBtn” element.
The function inside the event listener is doing three things:
- It is setting the
display
property of thepauseBtn
element to “block”, which will make thepauseBtn
element visible on the webpage. - It is setting the
display
property of theresumeBtn
element to “none”, which will hide theresumeBtn
element on the webpage. - It is checking if the
speechSynthesis.pause
is true, and if it is true, it’s using theresume
method of thespeechSynthesis
object to resume the speech synthesis.
So, in summary, when the user clicks on the “resumeBtn” element, the resumeBtn
will be hidden and the pauseBtn
will be shown and the speech synthesis will be resumed, assuming that it was paused previously.
resumeBtn.addEventListener("click", () => {
pauseBtn.style.display = "block";
resumeBtn.style.display = "none";
//resume the audio if it is paused
if (speechSynthesis.pause) {
speechSynthesis.resume();
}
});
This code is using the addEventListener
method to attach an event handler to the “pauseBtn” element. The event handler is a function that will be executed when the “click” event occurs on the “pauseBtn” element.
The function inside the event listener is doing three things:
- It is setting the
display
property of thepauseBtn
element to “none”, which will hide thepauseBtn
element on the webpage. - It is setting the
display
property of theresumeBtn
element to “block”, which will make theresumeBtn
element visible on the webpage. - It is checking if the
speechSynthesis.speaking
is true and if it is true it’s using thepause
method of thespeechSynthesis
object to pause the speech synthesis.
So, in summary, when the user clicks on the “pauseBtn” element, the pauseBtn
will be hidden and the resumeBtn
will be shown and the speech synthesis will be paused, assuming that it was speaking previously.
pause.addEventListener("click", () => {
pauseBtn.style.display = "none";
resumeBtn.style.display = "block";
//pause if speaking
speechSynthesis.speaking ? speechSynthesis.pause() : "";
});
This code defines an anonymous function that is executed when the window’s “load” event is fired. The function sets the display property of an element with the id “resumeBtn” to “none”, hiding it.
The function also checks if the browser supports the “speechSynthesis” object, and if it does, it creates a new instance of the “SpeechSynthesisUtterance” object, which is used for text-to-speech synthesis. If the browser does not support “speechSynthesis”, an alert is shown to the user.
window.onload = () => {
resumeBtn.style.display = "none";
if ("speechSynthesis" in window) {
audioMessage = new SpeechSynthesisUtterance();
} else {
alert("Speech Synthese is not supported");
}
};

I hope you got to know how I made it from the tutorial above. If you have any questions, you can comment.
I have already shared a tutorial on an advanced text-to-voice converter. If you have deep knowledge of JavaScript then you can follow that tutorial.
You can easily convert text to speech. But it won’t be possible with just html. You need to use javascript for this. To convert text to speech in HTML, you can use the SpeechSynthesis API. You can follow this tutorial.
There are several ways to automatically convert audio to text, also known as speech recognition or speech-to-text. Here are a few options:
Google Cloud Speech-to-Text API: This is a cloud-based service that allows you to transcribe audio files, including live speech. You can use the API to transcribe audio in over 120 languages and dialects.
CMU Sphinx: This is an open-source toolkit for speech recognition that can be used for both offline and real-time speech recognition.
Open Speech Recognition: This is a python package that allows you to perform speech recognition with the help of Google Speech Recognition service.
The Web Speech API is a browser-based API that allows developers to incorporate speech recognition and synthesis into web pages.
To convert text to voice using the Web Speech API, you can use the SpeechSynthesis interface, which allows you to control the speech synthesis engine. You can follow the tutorial above to learn more.
For this you can use libraries There are several libraries and frameworks that make it easy to implement text-to-speech (TTS) functionality in JavaScript. Here are a few popular options:
meSpeak.js: This is another JavaScript library that allows you to add TTS functionality to your web pages. It uses the eSpeak TTS engine and supports multiple languages.
SpeechKITT: This is a JavaScript library that allows you to add voice commands to your web pages using the Web Speech API. It has a simple API that can be used to create custom commands and responses.