Introduction:
This project is a simple music kit implemented using HTML, CSS, and JavaScript. It allows users to play different drum sounds by clicking on buttons or pressing corresponding keys on the keyboard. The HTML file provides the structure of the user interface, the CSS file styles the elements, and the JavaScript file handles the logic for playing sounds and highlighting the pressed buttons.
This project represents a dynamic and interactive music kit designed to engage users in creating rhythmic compositions effortlessly. Leveraging the power of web technologies like HTML, CSS, and JavaScript, it provides a seamless platform for users to explore their musical creativity.
Overall, the project serves as a testament to the boundless potential of web technologies in fostering creativity and innovation. Whether used as a standalone tool for musical experimentation or integrated into larger multimedia projects, the music kit represents a harmonious blend of artistry and technology, inviting users to embark on a journey of sonic exploration and self-expression
At its core, the project offers a visually intuitive interface comprising drum buttons, each mapped to a distinct percussive sound. Users can trigger these sounds either by clicking on the graphical buttons or by pressing corresponding keys on their keyboards, fostering a fluid and immersive user experience.
Through a combination of HTML for structure, CSS for styling, and JavaScript for interactivity, the project encapsulates the essence of web development, showcasing the synergy between design and functionality. This synergy not only facilitates seamless interaction but also ensures that users of all skill levels can effortlessly navigate and utilize the music kit to express themselves creatively.
By encapsulating audio files within a JavaScript module and dynamically associating them with button clicks or key presses, the project demonstrates the versatility and extensibility of modern web development techniques. This modular approach not only enhances maintainability but also allows for easy scalability, enabling the addition of new sounds or features with minimal effort.
Overall, the project serves as a testament to the boundless potential of web technologies in fostering creativity and innovation. Whether used as a standalone tool for musical experimentation or integrated into larger multimedia projects, the music kit represents a harmonious blend of artistry and technology, inviting users to embark on a journey of sonic exploration and self-expression.
Explanation :
HTML (index.html):
- The HTML file defines the structure of the web page.
- It includes references to the JavaScript file (
musickit.js
) and the CSS file (styles.css
). - Inside the
<body>
tag, there is a<div>
with the class “set”, which contains buttons representing different drum sounds.
CSS (styles.css):
- Defines the styles for the drum buttons and their hover effects.
- Each drum button has a specific background image and styling for its appearance.
- It also includes media queries for adjusting the layout on different screen sizes.
JavaScript (musickit.js):
- Imports audio files for each drum sound.
- Defines a map (
sounds
) that maps drum button characters to their corresponding audio files. - Adds event listeners to each drum button to play the associated sound when clicked.
- Listens for keypress events and plays the corresponding sound.
- Provides functions (
makeSound
andhighlightPressedButton
) to play the sound and highlight the pressed button, respectively.
JavaScript Logic:
- Imports: Import audio files for each drum sound.
- sounds Map: Maps drum button characters to their corresponding audio files.
- Event Listeners:
- Adds click event listeners to each drum button to play the associated sound and highlight the pressed button.
- Listens for keypress events to play the corresponding sound and highlight the pressed button.
- makeSound Function: Plays the sound associated with the pressed button.
- highlightPressedButton Function: Highlights the pressed button by adding the “pressed” class and removes the class from the previously pressed button.
At the heart of the project lies the JavaScript file, which orchestrates the interactive behavior of the music kit. Leveraging modern ES6 features such as modules and arrow functions, it encapsulates functionality in a modular and maintainable manner. Through the use of the import
statement, audio files for each drum sound are seamlessly integrated into the project, facilitating code organization and reuse. A Map
data structure is employed to establish a mapping between drum button characters and their corresponding audio files, enabling efficient retrieval and playback.
Event Handling:
The JavaScript file implements event listeners to capture user interactions and trigger appropriate responses. Click event listeners are attached to each drum button, allowing users to play sounds by clicking on the graphical interface. Similarly, a keypress event listener listens for keyboard input, enabling users to trigger sounds by pressing corresponding keys. This dual input mechanism enhances accessibility and user engagement, catering to diverse preferences and input modalities.
Functions and Modularity:
To promote code readability and maintainability, the JavaScript file defines reusable functions that encapsulate distinct pieces of functionality. The makeSound
function encapsulates the logic for playing audio files based on user input, facilitating code reuse and abstraction. Similarly, the highlightPressedButton
function manages the visual highlighting of pressed buttons, enhancing user feedback and interaction. By adopting a modular approach, the project exhibits best practices in software design, fostering extensibility and scalability.
In conclusion, the music kit project represents a harmonious fusion of design, functionality, and user experience, showcasing the transformative power of web technologies in fostering creativity and innovation. Through meticulous attention to detail, modular design principles, and a user-centric approach, the project exemplifies best practices in web development, serving as a testament to the limitless possibilities of digital expression and artistic exploration. Whether used as a standalone tool for musical experimentation or integrated into larger multimedia endeavors, the music kit embodies the ethos of web development as a catalyst for creativity and self-expression.
Purpose of Functions:
- makeSound: Plays the audio file associated with the pressed drum button or key.
- highlightPressedButton: Highlights the currently pressed button visually by adding a CSS class and removes the highlight from the previously pressed button.
SOURCE CODE :
HTML (index.html)
Music Kit
CSS (style.css)
.set {
display: flex;
justify-content: center;
align-items: center;
margin-top: 2rem;
flex-wrap: wrap;
}
.drum {
border: 2px solid #333;
background-color: #fff;
font-size: 1.5rem;
display: flex;
align-items: center;
justify-content: center;
cursor: pointer;
transition: background-color 0.2s, transform 0.2s;
border: 10px solid #404b69;
font-size: 5rem;
line-height: 2;
font-weight: 900;
color: #da0463;
text-shadow: 3px 0 #dbedf3;
width: 115px;
height: 115px;
border-radius: 15px;
margin: 10px;
}
/* Add hover effect to drum buttons */
.drum:hover {
background-color: #333;
color: blue;
transform: scale(1.1);
}
.w {
background-image: url('images/tom1.png');
}
.a {
background-image: url('images/tom2.png');
}
.s {
background-image: url('images/tom3.png');
}
.d {
background-image: url('images/tom4.png');
}
.j {
background-image: url('images/snare.png');
}
.k {
background-image: url('images/crash.png');
}
.l {
background-image: url('images/kick.png');
}
.pressed {
box-shadow: 0 3px 4px 0 #dbedf3;
opacity: 0.5;
}
.red {
color: red;
}
@media (width >=768px) {
.set {
margin: 10% auto;
}
.drum {
width: 150px;
height: 150px;
}
}
JavaScript ( musickit.js)
import tom1 from './sounds/tom-1.mp3';
import tom2 from './sounds/tom-2.mp3';
import tom3 from './sounds/tom-3.mp3';
import tom4 from './sounds/tom-4.mp3';
import snare from './sounds/snare.mp3';
import crash from './sounds/crash.mp3';
import kick from './sounds/kick-bass.mp3';
const sounds = new Map([
['w', tom1],
['a', tom2],
['s', tom3],
['d', tom4],
['j', snare],
['k', crash],
['l', kick],
]);
const numberOfDrumButtons = document.querySelectorAll('.drum').length;
for (let i = 0; i < numberOfDrumButtons; i++) {
document.querySelectorAll('.drum')[i].addEventListener('click', function () {
const buttonInnerHTML = this.textContent;
makeSound(buttonInnerHTML);
highlightPressedButton(buttonInnerHTML);
});
}
document.addEventListener('keypress', function (event) {
makeSound(event.key);
highlightPressedButton(event.key);
});
function makeSound(key) {
const audio = new Audio(sounds.get(key));
audio.play();
}
function highlightPressedButton(currentKey) {
const pressedButton = document.querySelector('.pressed');
if (pressedButton) {
pressedButton.classList.remove('pressed');
}
const activeButton = document.querySelector('.' + currentKey);
activeButton?.classList.add('pressed');
}
Download the images and sounds used in this project :
OUTPUT :
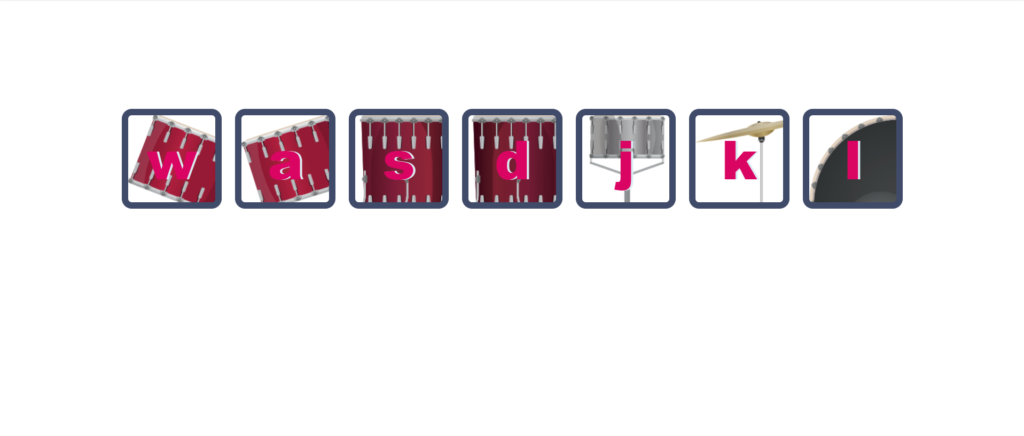