Introduction :
This Finance Tracker is a web application designed to help users manage their finances by keeping track of their transactions. It allows users to add both income and expense transactions, dynamically displaying them on the page along with their current balance.
It helps to empower individuals in managing their finances effectively. In an era where financial literacy and management are crucial, this tool serves as a beacon of assistance, providing a simple yet powerful platform for users to monitor their monetary inflows and outflows.
Financial management is not merely about tracking numbers; it’s about gaining control over one’s financial destiny. The Finance Tracker embodies this philosophy by offering a seamless interface that facilitates the recording and analysis of financial transactions. Whether it’s monitoring monthly expenses, tracking income sources, or identifying spending patterns, this tool equips users with the insights needed to make informed financial decisions.
Navigating through the complexities of personal finance can often be daunting. Recognizing this challenge, the Finance Tracker prioritizes user experience, offering an intuitive interface that simplifies the process of recording transactions. From adding income sources to categorizing expenses, every feature is meticulously crafted to ensure a seamless user journey, allowing individuals to focus on what matters most—taking control of their financial future.
Real-Time Insights:
In the fast-paced world of finance, having access to real-time information is paramount. The Finance Tracker empowers users with instant insights into their financial status, displaying their current balance alongside a detailed transaction history. By providing up-to-the-minute updates, users can stay informed about their financial health, enabling proactive decision-making and ensuring financial stability.
Customizable Financial Management:
Recognizing that every individual’s financial journey is unique, the Finance Tracker offers flexibility and customization options to cater to diverse needs. Whether it’s setting personalized budget goals, creating custom transaction categories, or generating tailored reports, users have the freedom to adapt the platform to suit their specific financial objectives, empowering them to chart their own path to financial success.
Commitment to Financial Wellness:
At its core, the Finance Tracker is more than just a tool; it’s a partner in the journey towards financial wellness. By promoting transparency, accountability, and mindfulness in financial management, this platform fosters a culture of empowerment and resilience, guiding users towards a brighter and more secure financial future.
In essence, the Finance Tracker is not just another application—it’s a catalyst for transformation, empowering individuals to take charge of their financial destiny and embark on a journey towards lasting prosperity and peace of mind.
Explanation :
The project consists of HTML, CSS, and JavaScript files. The HTML file defines the structure of the webpage, including sections for displaying the balance, adding transactions, and listing transactions. The CSS file styles the elements to enhance the visual appeal and readability of the webpage. The JavaScript file provides the logic for updating the balance, adding transactions, and validating user inputs.
HTML Structure:
- The HTML file provides the structural foundation of the Finance Tracker web application.
- It comprises several sections, each serving a distinct purpose:
- Balance Section: Displays the current balance.
- Add Transaction Section: Allows users to input transaction details such as description, amount, and type (income or expense).
- Transactions Section: Lists the recorded transactions.
- Each section is semantically defined using appropriate HTML5 tags, enhancing accessibility and SEO-friendliness.
CSS Styling:
- The CSS file styles the HTML elements to enhance visual appeal and user experience.
- It employs a combination of layout, typography, color, and animation techniques to create a cohesive and aesthetically pleasing design.
- Selectors target specific elements, applying styles such as colors, margins, padding, borders, and animations to achieve the desired presentation.
- CSS animations add dynamic visual effects, such as background color changes and item fade-ins, improving interactivity and engagement.
JavaScript Logic:
- The JavaScript file provides the functional logic required for the Finance Tracker’s dynamic behavior.
- It initializes variables to reference important DOM elements such as the balance amount, transaction list, and user input fields.
- Functions are defined to handle key tasks:
- updateBalance(): Updates the displayed balance based on recorded transactions.
- addTransaction(description, amount, type): Adds a new transaction to the list and updates the balance accordingly.
- Event listeners are set up to respond to user interactions, such as clicking the add transaction button.
- Input validation ensures that only valid transaction data is processed, enhancing data integrity and user experience.
Purpose of Functions:
- updateBalance(): Ensures that the balance displayed to the user accurately reflects the total of all recorded transactions. It maintains data consistency and provides users with real-time financial information.
- addTransaction(description, amount, type): Facilitates the addition of new transactions to the list, updating both the displayed transactions and the overall balance. This function encapsulates the transactional logic, making the addition process modular and reusable.
- Event listeners: Enable the Finance Tracker to respond to user actions in an interactive manner. They trigger appropriate functions based on user inputs, driving the dynamic behavior of the application.
- Input validation: Enhances the robustness of the application by ensuring that only valid transaction data is processed. This prevents errors and inconsistencies, maintaining the integrity of the financial records.
In-depth Analysis:
- The HTML, CSS, and JavaScript files work synergistically to create a cohesive and functional web application.
- The HTML defines the structure, the CSS styles the presentation, and the JavaScript provides the dynamic behavior.
- Modularization and encapsulation principles are observed in the JavaScript code, promoting code reusability, maintainability, and scalability.
- User experience considerations, such as animation effects and input validation, enhance usability and engagement.
- The application’s architecture reflects best practices in web development, fostering a robust and user-friendly financial management solution.
SOURCE CODE :
HTML (index.html)
Finance Tracker
Your Balance: $0.00
Add Transaction
Transactions
CSS (style.css)
* {
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
}
header {
background-color: #333;
color: #fff;
text-align: center;
padding: 1rem 0;
animation: headerBgChange 4s infinite alternate;
}
/* Animate header background color */
@keyframes headerBgChange {
0% {
background-color: #333;
}
100% {
background-color: #007bff;
}
}
header h1 {
margin: 0;
}
main {
max-width: 800px;
margin: 0 auto;
padding: 1rem;
background-color: #fff;
border-radius: 5px;
}
#balance {
text-align: center;
}
#balance h2 {
margin: 0;
font-size: 1.5rem;
}
#balance-amount {
color: #28a745;
}
#transactions h2,
#add-transaction h2 {
font-size: 1.2rem;
margin-top: 1rem;
}
ul {
list-style: none;
padding: 0;
}
li {
display: flex;
justify-content: space-between;
margin: 0.5rem 0;
padding: 0.5rem;
border: 1px solid #ccc;
background-color: #fff;
border-radius: 5px;
animation: listItemFadeIn 0.5s ease-in-out;
}
/* Animate transaction list items */
@keyframes listItemFadeIn {
0% {
opacity: 0;
transform: translateY(-10px);
}
100% {
opacity: 1;
transform: translateY(0);
}
}
.income {
color: #28a745;
}
.expense {
color: #dc3545;
}
label,
input,
select,
button {
display: block;
margin-bottom: 0.5rem;
}
input[type='text'],
input[type='number'],
select {
width: 100%;
padding: 0.5rem;
border: 1px solid #ccc;
border-radius: 5px;
}
button {
background-color: #007bff;
color: #fff;
border: none;
padding: 0.5rem 1rem;
border-radius: 5px;
cursor: pointer;
}
button:hover {
background-color: #0056b3;
}
button:hover {
background-color: #0056b3;
transform: scale(1.05);
transition: background-color 0.3s, transform 0.3s;
}
JavaScript (index.js)
const balanceAmount = document.getElementById('balance-amount');
const transactionList = document.getElementById('transaction-list');
const descriptionInput = document.getElementById('description');
const amountInput = document.getElementById('amount');
const transactionTypeInput = document.getElementById('transaction-type');
const addButton = document.getElementById('add-button');
let balance = 0;
function updateBalance() {
balanceAmount.textContent = `$${balance.toFixed(2)}`;
}
function addTransaction(description, amount, type) {
const transaction = document.createElement('li');
transaction.classList.add(type);
transaction.innerHTML = `
${description}
$${amount.toFixed(2)}
`;
transactionList.appendChild(transaction);
balance += type === 'income' ? amount : -amount;
updateBalance();
}
addButton.addEventListener('click', () => {
const description = descriptionInput.value;
const amount = parseFloat(amountInput.value);
const type = transactionTypeInput.value;
if (description.trim() === '' || isNaN(amount) || amount <= 0) {
alert('Please enter a valid description and amount.');
return;
}
addTransaction(description, amount, type);
descriptionInput.value = '';
amountInput.value = '';
});
// Initialize balance
updateBalance();
OUTPUT :
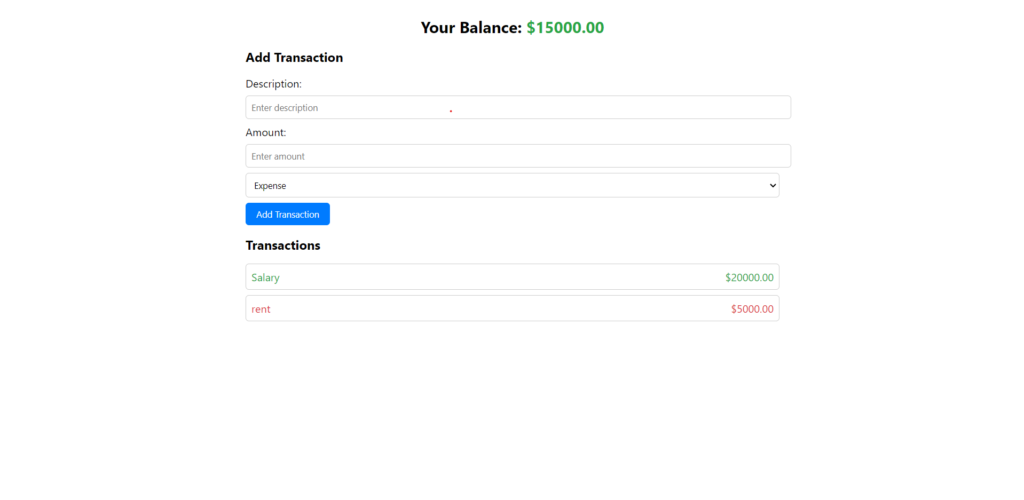