Introduction :
This bill splitting website aims to simplify the process of dividing a bill among a group of people. It allows users to input the total bill amount, select a tip percentage, and specify the number of people sharing the bill. Based on these inputs, the website calculates the tip amount, total amount to be paid, and each person’s share of the bill. Additionally, it provides functionality to reset the inputs and start over.
This bill splitting website serves as a practical solution for individuals or groups facing the common challenge of dividing expenses evenly among multiple parties. With its intuitive interface and robust functionality, it simplifies the intricate process of splitting bills, making it an indispensable tool for social gatherings, business meetings, or any situation involving shared expenses.
Imagine a scenario where friends dine out together, each ordering their favorite dishes and drinks. As the meal concludes, the inevitable question arises: “How do we split the bill?” This seemingly simple query can quickly become a logistical nightmare, especially when considering varying meal costs, differing tipping preferences, and the number of individuals involved. Here, this website steps in, transforming the tedious task of bill division into a streamlined and hassle-free experience.
Structural Foundation:
At its core, the website relies on a solid structural foundation provided by HTML (Hypertext Markup Language). HTML elements such as containers, labels, and input fields are meticulously organized to create an intuitive user interface. Each element serves a distinct purpose, contributing to the overall functionality and aesthetic appeal of the website.
Enhancement:
Enhancing the user experience is the CSS (Cascading Style Sheets) styling, which adds visual flair and cohesiveness to the website. Through carefully crafted style rules, elements are adorned with captivating colors, elegant fonts, and responsive design, ensuring a visually appealing presentation across various devices and screen sizes.
Interactive Dynamics:
However, it is the dynamic interactivity facilitated by JavaScript that truly sets this website apart. JavaScript functions orchestrate user interactions, validate input data, perform real-time calculations, and update the display accordingly. Whether selecting tip percentages, inputting custom tips, specifying the number of people, or generating the final bill, JavaScript seamlessly orchestrates every step of the process with precision and efficiency.
Functional Core:
The heart of the website lies in its JavaScript logic, where a series of meticulously crafted functions govern every aspect of bill splitting. From input validation to result calculation, each function plays a vital role in ensuring accuracy and reliability. Whether it’s enabling/disabling buttons based on input validity, calculating tip amounts, or resetting the interface to its initial state, these functions work in harmony to deliver a seamless user experience.
Practical Utility:
Beyond its technical intricacies, this website embodies practical utility, offering a tangible solution to a ubiquitous problem. Whether it’s a casual dinner with friends, a business lunch, or a group outing, the website empowers users to effortlessly divide expenses, fostering harmony and convenience in shared financial transactions.
In essence, this bill splitting website transcends mere digital functionality, embodying the ethos of simplicity, efficiency, and practicality. By seamlessly integrating HTML, CSS, and JavaScript, it transforms the complex chore of bill division into a seamless and enjoyable experience, epitomizing the power of technology to simplify everyday tasks and enhance social interactions.
Explanation :
The website consists of three main sections: HTML for structure and content, CSS for styling, and JavaScript for interactivity and functionality.
HTML Structure:
The HTML structure defines the layout of the website, including input fields, buttons, and result displays. It uses semantic tags like <div>
, <label>
, and <input>
for structuring the content logically. The layout is divided into two main sections: left section for input fields and right section for displaying calculated results.
CSS Styling:
The CSS styling provides visual enhancements to the website, including colors, fonts, spacing, and responsiveness for different screen sizes. It styles various elements such as containers, input fields, buttons, and result displays to create an aesthetically pleasing and user-friendly interface.
JavaScript Logic:
The JavaScript logic handles user interactions and performs calculations based on input values. It consists of several functions to validate input, calculate tip and total amounts, update display, and handle reset functionality.
validateInput()
: Checks if all required input fields have valid values and enables/disables the “Generate Bill” button accordingly.validateBill()
: Validates the bill amount input field and enables/disables tip buttons, custom tip input, and number of people input accordingly.selectTip(e)
: Handles the selection of tip percentage buttons and calculates the tip amount accordingly. It also enables/disables the custom tip input field.tipCustomValue()
: Handles the input of custom tip percentage and calculates the tip amount accordingly.setPeopleValue()
: Handles the input of the number of people sharing the bill.calculate()
: Calculates the tip amount, total amount to be paid, and each person’s share of the bill based on input values.handleReset()
: Resets all input fields, disables unnecessary elements, and clears calculated results when the reset button is clicked.
Purpose of Functions:
- Validation Functions (
validateInput()
,validateBill()
): Ensure that all required input fields have valid values before allowing the user to generate the bill. - Tip Selection Functions (
selectTip(e)
,tipCustomValue()
): Handle the selection of tip percentage either through predefined buttons or custom input. - Input Handling Functions (
setPeopleValue()
): Handle the input of the number of people sharing the bill. - Calculation Function (
calculate()
): Calculate tip amount, total amount, and each person’s share of the bill based on input values. - Reset Function (
handleReset()
): Reset all input fields and clear calculated results to start over.
These functions collectively provide the core functionality of the bill splitting website, ensuring a seamless and efficient user experience.
SOURCE CODE :
HTML (index.html)
Bill Splitter
₹
Tip amount
Total
Each Person Bill
CSS (style.css)
.bill-container {
margin: auto;
display: flex;
background-color: #fff;
justify-content: space-between;
border-radius: 10px;
max-width: 700px;
gap: 20px;
margin-top: 20px;
}
.inside_left {
width: 100%;
display: flex;
flex-direction: column;
gap: 20px;
}
.input-container {
display: flex;
flex-direction: column;
gap: 10px;
}
.input-container>label {
color: #6f3cff;
font-size: 20px;
}
.amount-container {
display: flex;
width: 100%;
height: 35px;
padding: 10px;
align-items: center;
font-size: 20px;
gap: 10px;
border: 1px solid black;
border-radius: 4px;
color: #6f3cff;
}
.amount-container>input {
border: none;
outline: none;
width: 100%;
height: 30px;
background-color: transparent;
font-size: 20px;
color: #6f3cff;
}
input[type='number']::-webkit-inner-spin-button,
input[type='number']::-webkit-outer-spin-button {
-webkit-appearance: none;
-moz-appearance: none;
appearance: none;
margin: 0;
}
.tip-container {
display: flex;
flex-direction: column;
gap: 10px;
}
.tip-container>label {
color: #6f3cff;
font-size: 20px;
}
.btn-tip {
display: grid;
grid-template-columns: repeat(auto-fill, minmax(70px, 1fr));
gap: 15px;
}
.customTip {
width: 100%;
}
.customTip>input {
width: 100%;
height: 35px;
padding: 10px;
font-size: 15px;
outline: none;
border: none;
background-color: #ccc;
color: #6f3cff;
}
.btn {
padding: 10px;
border: none;
outline: none;
border-radius: 5px;
font-size: 20px;
}
.btn.true {
background-color: #9c60ff;
color: #fff;
cursor: pointer;
}
.btn.active {
outline: 4px solid rgba(255, 162, 0, 0.888);
}
.people-container {
display: flex;
flex-direction: column;
gap: 10px;
}
.people-container>label {
color: #6f3cff;
font-size: 20px;
}
.people-container>input {
width: 100%;
height: 35px;
padding: 10px;
font-size: 15px;
outline: none;
border: none;
background-color: #ccc;
color: #6f3cff;
}
.generate {
width: 100%;
height: 40px;
border: none;
font-size: 15px;
border-radius: 7px;
cursor: not-allowed;
}
.generate.active {
background-color: #9c60ff;
color: white;
cursor: pointer;
}
.inside_right {
background: #34568b;
width: 100%;
display: flex;
margin: 30px 0 20px 0;
flex-direction: column;
justify-content: space-between;
padding: 30px;
border-radius: 10px;
}
.total-amount {
display: flex;
flex-direction: column;
gap: 20px;
}
.tip-amount {
display: flex;
justify-content: space-between;
align-items: center;
}
.tip-amount>h4 {
font-size: 16px;
color: #fff;
}
.tip-amount>span {
font-size: 25px;
color: #c39fff;
font-weight: 700;
}
.total {
display: flex;
justify-content: space-between;
align-items: center;
}
.total>h4 {
font-size: 16px;
color: #fff;
}
.total>span {
font-size: 25px;
color: #c39fff;
font-weight: 700;
}
#reset {
padding: 12px;
border: none;
border-radius: 5px;
font-size: 16px;
width: 100%;
cursor: not-allowed;
}
#reset.active {
background-color: #ffffff;
color: black;
cursor: pointer;
}
@media only screen and (max-width: 600px) {
.bill-container {
flex-direction: column;
}
.total-amount {
gap: 10px;
}
.btn-tip {
grid-template-columns: repeat(auto-fill, minmax(100px, 1fr));
}
}
JavaScript (index.js)
const amount = document.getElementById('amount');
const tipButton = document.querySelectorAll('.btn');
const customTip = document.getElementById('customTip');
const noOfPeople = document.getElementById('person');
const generateBill = document.getElementById('generate-bill');
const tipValue = document.querySelector('.tipValue');
const totalValue = document.querySelector('.totalValue');
const eachPersonBill = document.querySelector('.bill');
const resetBtn = document.getElementById('reset');
let billValue = 0;
let tip = 0;
let people = 0;
function validateInput() {
if (billValue > 0 && tip > 0 && people > 0) {
generateBill.classList.add('active');
generateBill.disabled = false;
return true;
} else {
generateBill.classList.remove('active');
generateBill.disabled = true;
return false;
}
}
function validateBill() {
billValue = parseFloat(amount.value);
tipButton.forEach((btn) => {
if (billValue > 0) {
btn.classList.add('true');
btn.disabled = false;
customTip.disabled = false;
noOfPeople.disabled = false;
} else {
btn.classList.remove('true');
btn.disabled = true;
customTip.disabled = true;
noOfPeople.disabled = true;
}
});
validateInput();
}
function selectTip(e) {
tipButton.forEach((btn) => {
btn.classList.remove('active');
if (e && e.target.innerHTML == btn.innerHTML) {
btn.classList.add('active');
tip = parseFloat(btn.innerHTML) / 100;
}
});
customTip.value = '';
validateInput();
}
function tipCustomValue() {
if (customTip.value !== 0) {
tip = parseFloat(customTip.value / 100);
tipButton.forEach((btn) => {
btn.classList.remove('active');
});
}
validateInput();
}
function setPeopleValue() {
people = parseFloat(noOfPeople.value);
validateInput();
}
function calculate() {
if (people >= 1) {
let tipAmount = billValue * tip;
let totalAmount = billValue + tipAmount;
let divideBill = totalAmount / people;
tipValue.innerHTML = '₹' + tipAmount.toFixed(2);
totalValue.innerHTML = '₹' + totalAmount.toFixed(2);
eachPersonBill.innerHTML = '₹' + divideBill.toFixed(2);
resetBtn.classList.add('active');
resetBtn.disabled = false;
}
}
function handleReset() {
amount.value = '';
validateBill();
tipButton.forEach((btn) => {
btn.classList.remove('true');
btn.disabled = true;
});
tip = '';
customTip.value = '';
selectTip();
noOfPeople.value = '';
setPeopleValue();
validateInput();
generateBill.disabled = true
tipValue.innerHTML = '';
totalValue.innerHTML = '';
eachPersonBill.innerHTML = '';
resetBtn.classList.remove('active');
resetBtn.disabled = true;
}
amount.addEventListener('input', validateBill);
generateBill.addEventListener('click', calculate);
customTip.addEventListener('input', tipCustomValue);
noOfPeople.addEventListener('input', setPeopleValue);
resetBtn.addEventListener('click', handleReset);
tipButton.forEach((btn) => {
btn.addEventListener('click', selectTip);
});
OUTPUT :
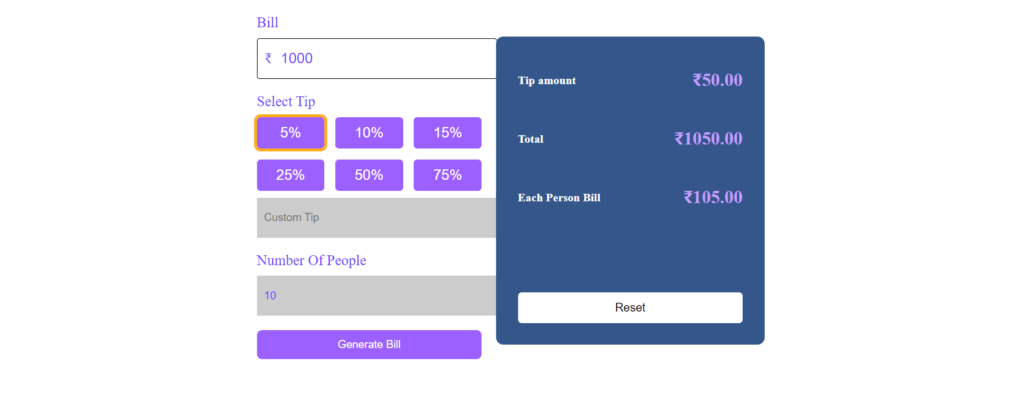